标签:box rotate candies length base board row
1861. Rotating the Box Medium You are given anm x n
matrix of characters box
representing a side-view of a box. Each cell of the box is one of the following:
- A stone
'#'
- A stationary obstacle
'*'
- Empty
'.'
The box is rotated 90 degrees clockwise, causing some of the stones to fall due to gravity. Each stone falls down until it lands on an obstacle, another stone, or the bottom of the box. Gravity does not affect the obstacles' positions, and the inertia from the box's rotation does not affect the stones' horizontal positions.
It is guaranteed that each stone in box
rests on an obstacle, another stone, or the bottom of the box.
Return an n x m
matrix representing the box after the rotation described above.
Example 1:
Input: box = [["#",".","#"]] Output: [["."], ["#"], ["#"]]
Example 2:
Input: box = [["#",".","*","."], ["#","#","*","."]] Output: [["#","."], ["#","#"], ["*","*"], [".","."]]
Example 3:
Input: box = [["#","#","*",".","*","."], ["#","#","#","*",".","."], ["#","#","#",".","#","."]] Output: [[".","#","#"], [".","#","#"], ["#","#","*"], ["#","*","."], ["#",".","*"], ["#",".","."]]
Constraints:
m == box.length
n == box[i].length
1 <= m, n <= 500
box[i][j]
is either'#'
,'*'
, or'.'
.
class Solution { public char[][] rotateTheBox(char[][] box) { for(int i=0;i<box.length;i++){ rotateRow(box[i]); } //向右翻转矩阵 char[][] result = new char[box[0].length][box.length]; for(int i=0;i<box.length;i++){ for(int j=0;j<box[0].length;j++){ //之前的第一行为现在的最后一列,之前的第一列为现在的第一行 //因此: 列转行 j->j 行转列 i->len-i-1 result[j][box.length-1-i]=box[i][j]; } } return result; } private void rotateRow(char[] row){ int base = row.length-1; for(int i=row.length-1;i>=0;i--){ //如果遇到障碍物 if(row[i]=='*') { //base向上移动 清空,直至碰到障碍物 while(base>i ) row[base--]='.'; //将新base置为障碍物之上的第一个位置 base=i-1; } //如果是石头,往base处进行填充 else if(row[i]=='#') row[base--]=row[i]; } while( base>=0 ) row[base--]='.'; } }723. Candy Crush Medium
This question is about implementing a basic elimination algorithm for Candy Crush.
Given an m x n
integer array board
representing the grid of candy where board[i][j]
represents the type of candy. A value of board[i][j] == 0
represents that the cell is empty.
The given board represents the state of the game following the player's move. Now, you need to restore the board to a stable state by crushing candies according to the following rules:
- If three or more candies of the same type are adjacent vertically or horizontally, crush them all at the same time - these positions become empty.
- After crushing all candies simultaneously, if an empty space on the board has candies on top of itself, then these candies will drop until they hit a candy or bottom at the same time. No new candies will drop outside the top boundary.
- After the above steps, there may exist more candies that can be crushed. If so, you need to repeat the above steps.
- If there does not exist more candies that can be crushed (i.e., the board is stable), then return the current board.
You need to perform the above rules until the board becomes stable, then return the stable board.
Example 1:
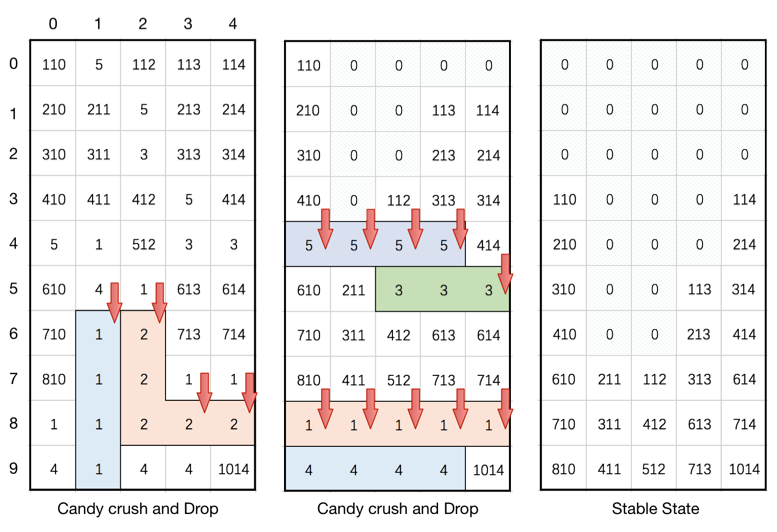
Input: board = [[110,5,112,113,114],[210,211,5,213,214],[310,311,3,313,314],[410,411,412,5,414],[5,1,512,3,3],[610,4,1,613,614],[710,1,2,713,714],[810,1,2,1,1],[1,1,2,2,2],[4,1,4,4,1014]] Output: [[0,0,0,0,0],[0,0,0,0,0],[0,0,0,0,0],[110,0,0,0,114],[210,0,0,0,214],[310,0,0,113,314],[410,0,0,213,414],[610,211,112,313,614],[710,311,412,613,714],[810,411,512,713,1014]]
Example 2:
Input: board = [[1,3,5,5,2],[3,4,3,3,1],[3,2,4,5,2],[2,4,4,5,5],[1,4,4,1,1]] Output: [[1,3,0,0,0],[3,4,0,5,2],[3,2,0,3,1],[2,4,0,5,2],[1,4,3,1,1]]
Constraints:
m == board.length
n == board[i].length
3 <= m, n <= 50
1 <= board[i][j] <= 2000
class Solution { public int[][] candyCrush(int[][] board) { //while loop while(true){ if(crush(board)) falldown(board); else break; } return board; } private boolean crush(int[][] board){ boolean flag=false; int m=board.length,n=board[0].length; //判定横向相连的 for(int i=0;i<m;i++){ for(int j=0;j<n-2;j++){ int curr = Math.abs(board[i][j]); if(curr==Math.abs(board[i][j+1]) && curr==Math.abs(board[i][j+2]) && curr!=0){ board[i][j]=board[i][j+1]=board[i][j+2]=-curr; flag=true; } } } //判定纵向相连的 for(int i=0;i<m-2;i++){ for(int j=0;j<n;j++){ int curr = Math.abs(board[i][j]); if(curr==Math.abs(board[i+1][j]) && curr==Math.abs(board[i+2][j]) && curr!=0){ board[i][j]=board[i+1][j]=board[i+2][j]=-curr; flag=true; } } } return flag; } private void falldown(int[][] board){ int row=board.length,col=board[0].length; for(int i=0;i<col;i++){ //双指针,base指向第一个不为0的数,j指向当前遍历元素 int base=row-1; for(int j=row-1;j>=0;j--){ if(board[j][i]>0) board[base--][i]=board[j][i]; } //当j指针到达最上面以后,base以上的位置都可以置0了 while(base>=0){ board[base--][i]=0; } } } }
标签:box,rotate,candies,length,base,board,row 来源: https://www.cnblogs.com/cynrjy/p/15926327.html
本站声明: 1. iCode9 技术分享网(下文简称本站)提供的所有内容,仅供技术学习、探讨和分享; 2. 关于本站的所有留言、评论、转载及引用,纯属内容发起人的个人观点,与本站观点和立场无关; 3. 关于本站的所有言论和文字,纯属内容发起人的个人观点,与本站观点和立场无关; 4. 本站文章均是网友提供,不完全保证技术分享内容的完整性、准确性、时效性、风险性和版权归属;如您发现该文章侵犯了您的权益,可联系我们第一时间进行删除; 5. 本站为非盈利性的个人网站,所有内容不会用来进行牟利,也不会利用任何形式的广告来间接获益,纯粹是为了广大技术爱好者提供技术内容和技术思想的分享性交流网站。